Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1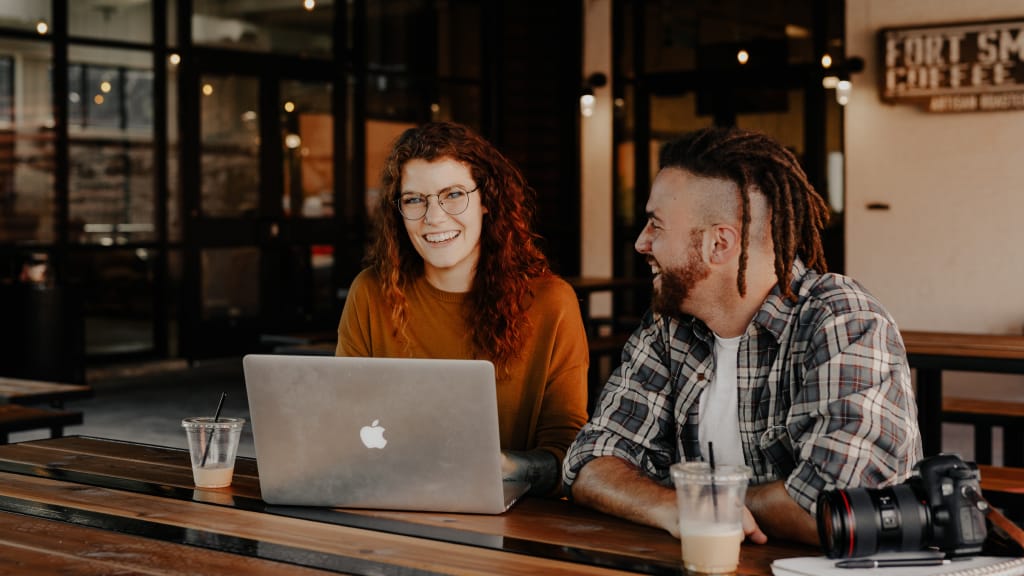
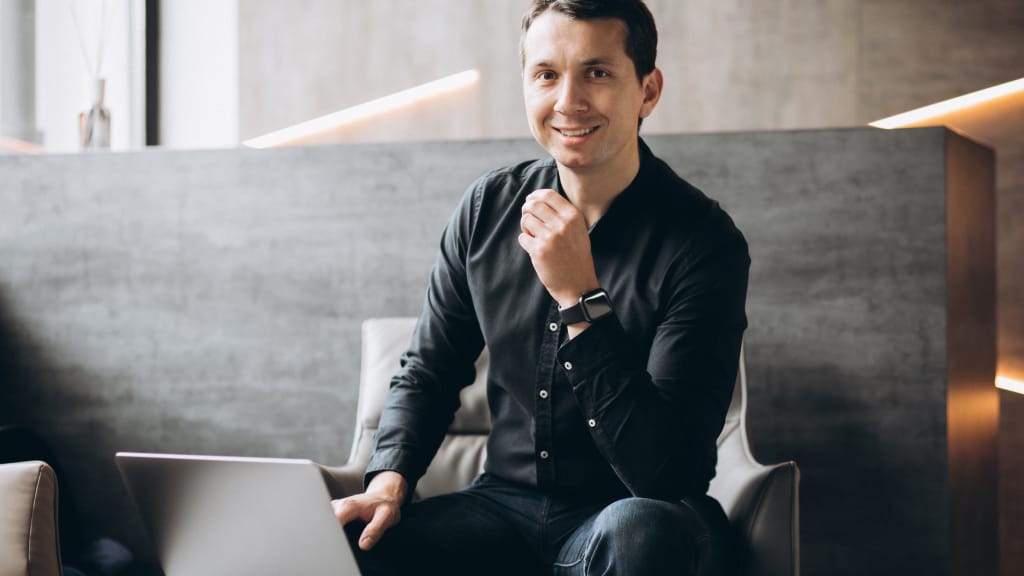
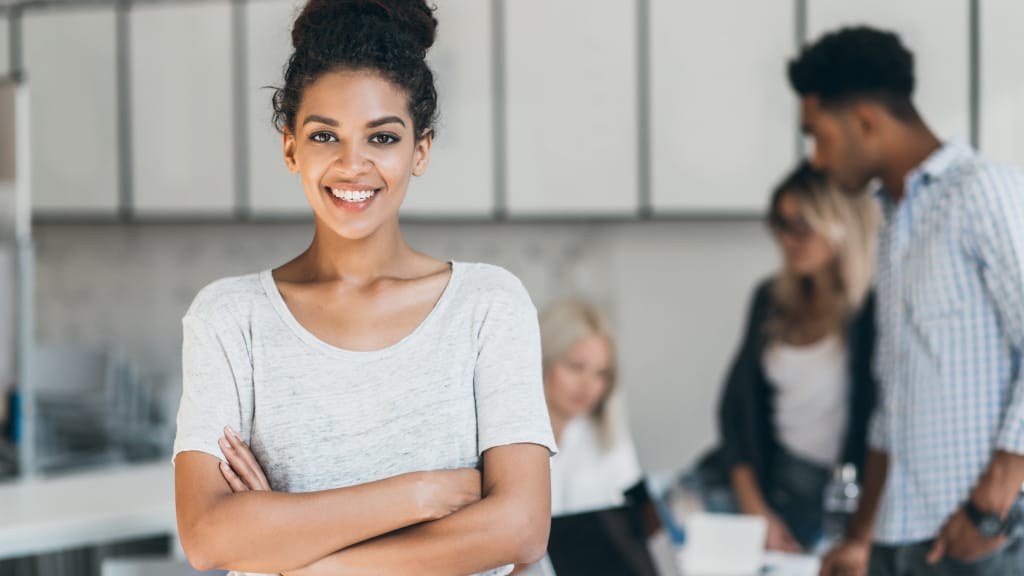
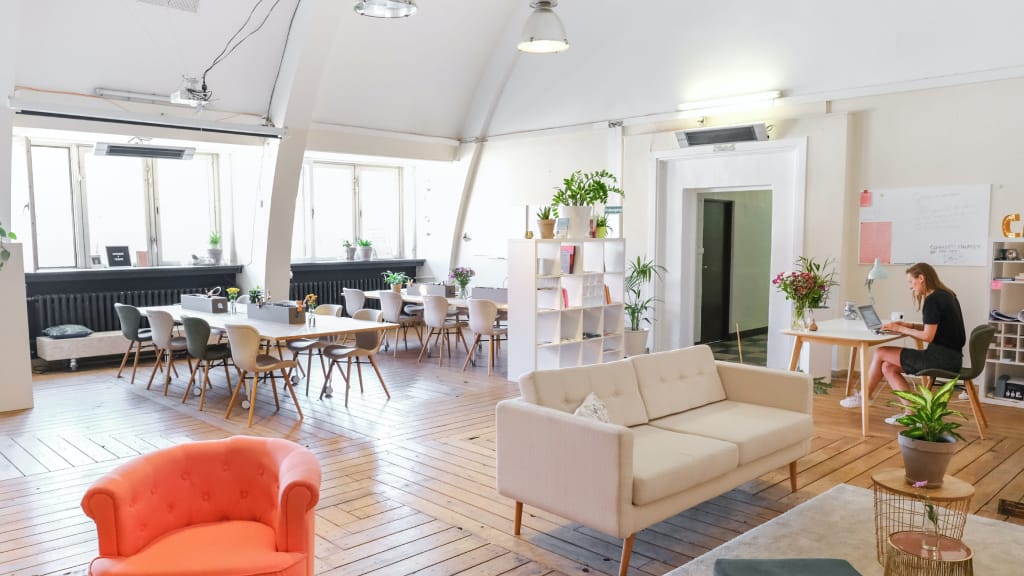
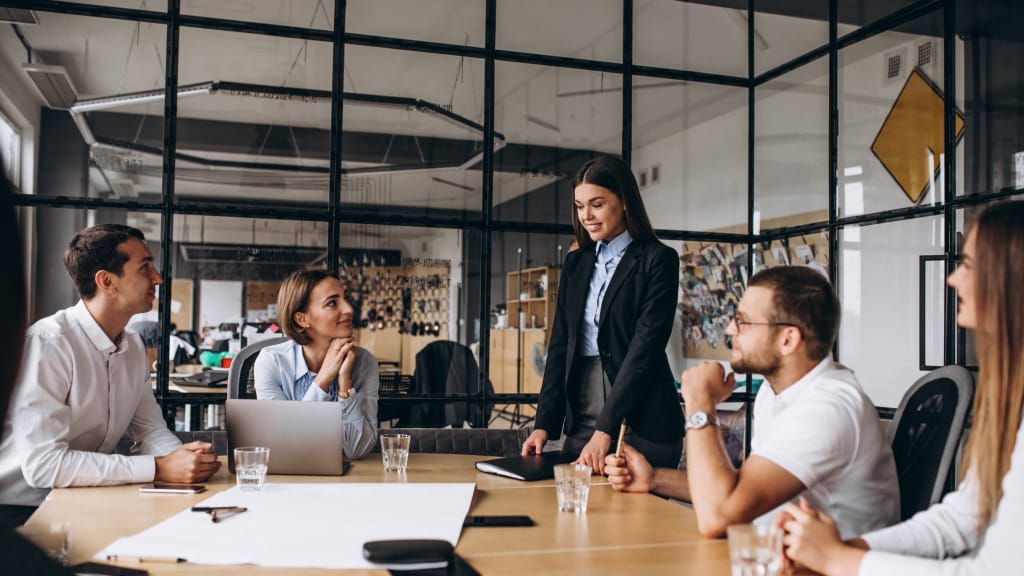
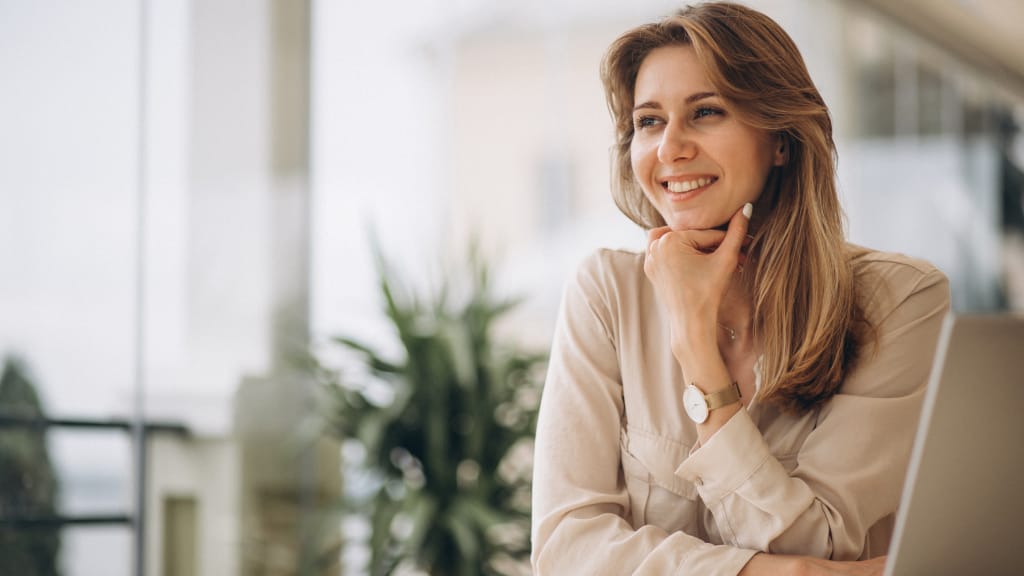
C++ File Streaming
In C++, file streaming allows you to read from and write to files using stream objects. The C++ standard library provides the <iostream>
header, which includes the necessary classes and functions for file input/output operations. In this article, we will explore file streaming in C++ with examples.
1. Writing to a File
To write data to a file in C++, you can use an output file stream object, such as std::ofstream
. Here's an example:
#include <iostream>
#include <fstream>
int main() {
std::ofstream outputFile("output.txt");
if (outputFile.is_open()) {
outputFile << "Hello, World!" << std::endl;
outputFile << "This is a line of text." << std::endl;
outputFile.close();
} else {
std::cout << "Failed to open the file." << std::endl;
}
return 0;
}
In the above code, we create an output file stream object named outputFile
and open a file named output.txt
. We use the stream insertion operator (<<
) to write data to the file. After writing, we close the file using the close()
function. If the file fails to open, we output an error message.
2. Reading from a File
To read data from a file in C++, you can use an input file stream object, such as std::ifstream
. Here's an example:
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream inputFile("input.txt");
if (inputFile.is_open()) {
std::string line;
while (std::getline(inputFile, line)) {
std::cout << line << std::endl;
}
inputFile.close();
} else {
std::cout << "Failed to open the file." << std::endl;
}
return 0;
}
In the above code, we create an input file stream object named inputFile
and open a file named input.txt
. We use the getline()
function to read each line of the file and output it to the console. After reading, we close the file. If the file fails to open, we output an error message.
3. Appending to a File
If you want to append data to an existing file without overwriting its contents, you can use the std::ofstream
object with the std::ios::app
flag. Here's an example:
#include <iostream>
#include <fstream>
int main() {
std::ofstream outputFile("data.txt", std::ios::app);
if (outputFile.is_open()) {
outputFile << "New data" << std::endl;
outputFile.close();
} else {
std::cout << "Failed to open the file." << std::endl;
}
return 0;
}
In the above code, we open the file data.txt
in append mode by passing the std::ios::app
flag to the std::ofstream
constructor. This ensures that the new data is appended to the existing content of the file instead of overwriting it. We then write the new data to the file and close it. If the file fails to open, we output an error message.
File streaming in C++ provides a convenient way to read from and write to files. Whether you need to save data to a file or retrieve data from it, file streaming allows you to handle file input/output operations efficiently in your C++ programs.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses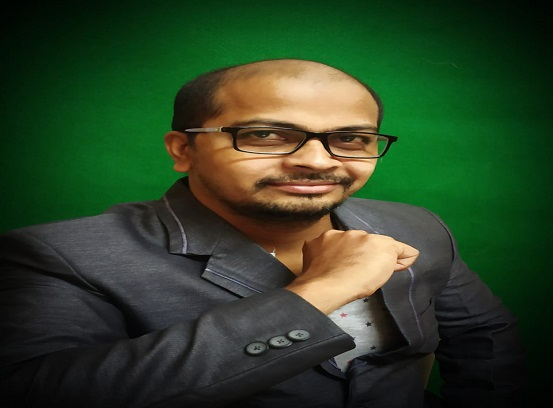
Most Popular Course topics
These are the most popular course topics among Software Courses for learners