Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1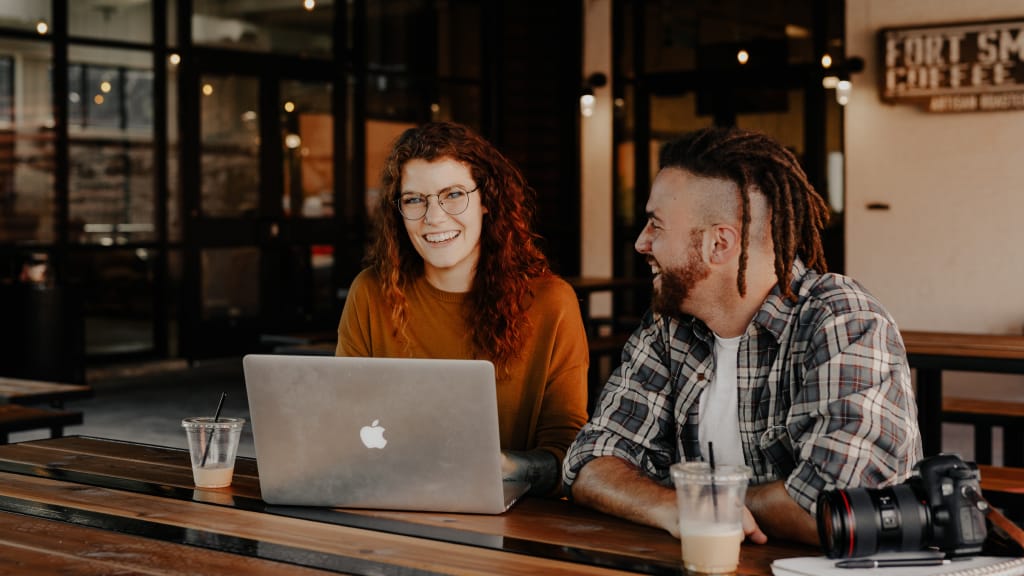
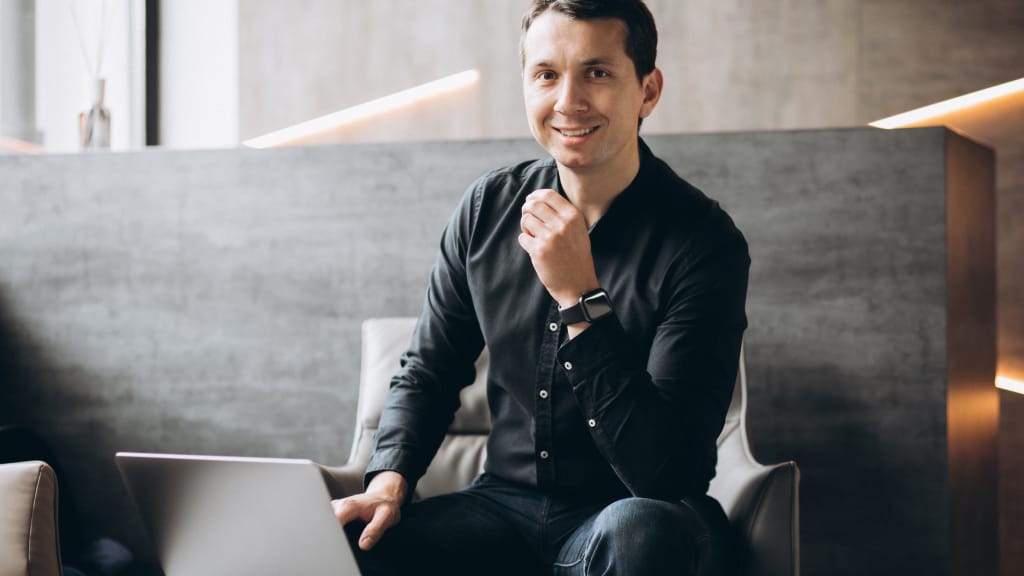
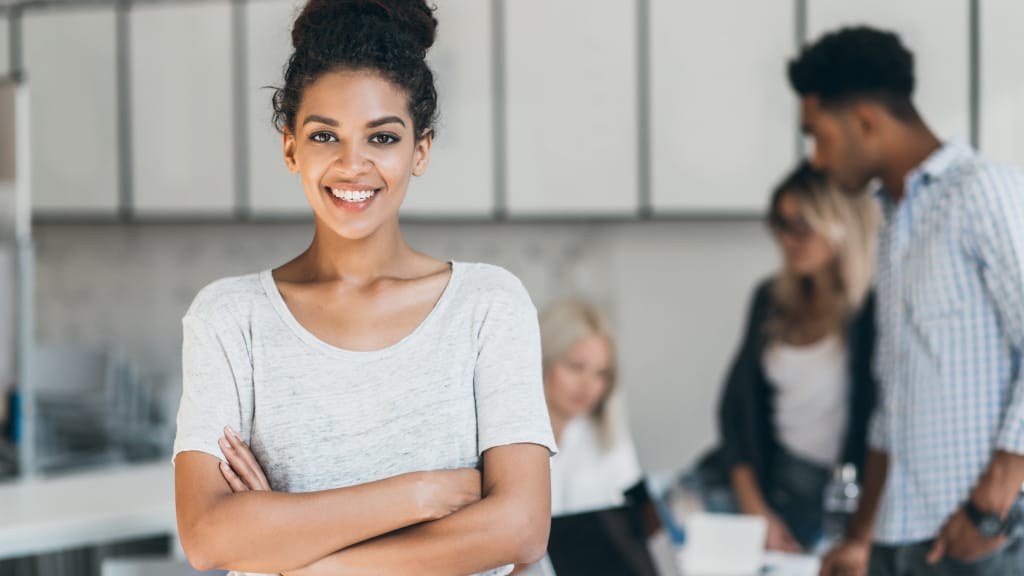
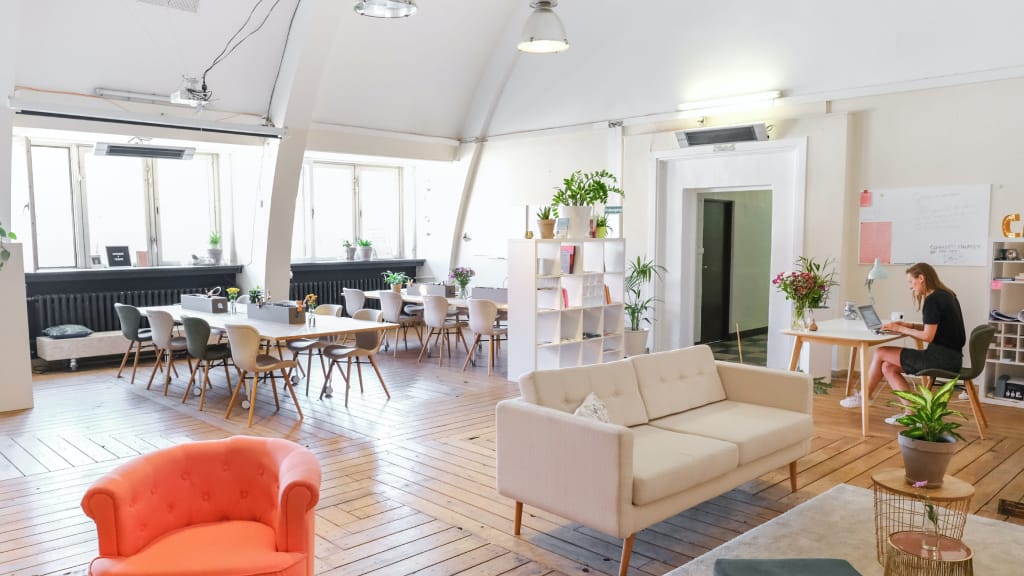
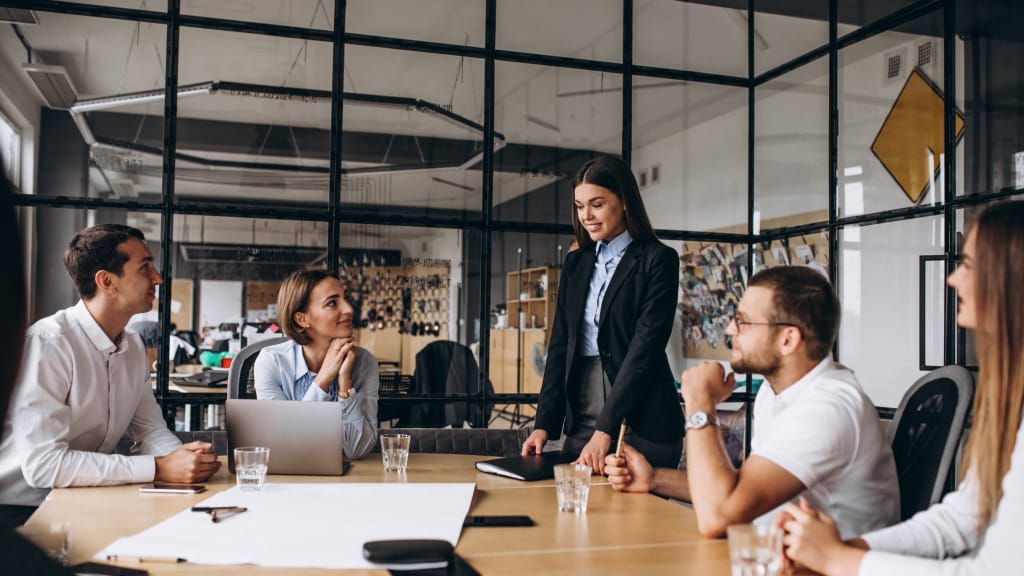
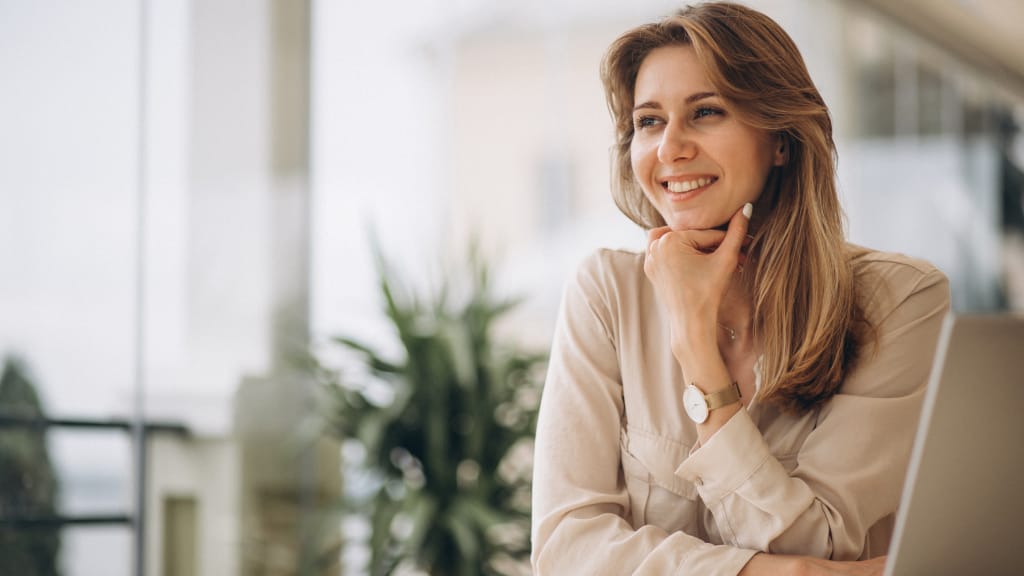
Python While Loop
The while loop is a control structure in Python that allows you to repeatedly execute a block of code as long as a certain condition is true. It is used when you want to repeat a set of instructions until the specified condition becomes false. Let's explore the syntax and usage of the while loop in Python with examples.
Syntax
The basic syntax of a while loop in Python is as follows:
while condition:
# Code to execute while the condition is true
Example 1: Printing Numbers
Consider the following example:
num = 1
while num <= 5:
print(num)
num += 1
In this example, we initialize the variable "num" to 1. The while loop continues executing as long as the condition "num <= 5" is true. Inside the loop, we print the current value of "num" and then increment it by 1. The loop repeats until the value of "num" becomes 6, at which point the condition becomes false, and the loop terminates.
Example 2: Summing Numbers
You can also use a while loop to perform calculations. Here's an example that calculates the sum of numbers from 1 to 10:
num = 1
sum = 0
while num <= 10:
sum += num
num += 1
print("The sum is:", sum)
In this example, we initialize "num" to 1 and "sum" to 0. Inside the while loop, we add the current value of "num" to "sum" and then increment "num" by 1. The loop continues until "num" reaches 11. After the loop terminates, we print the final value of "sum," which is the sum of numbers from 1 to 10.
Example 3: User Input
You can use a while loop to repeatedly prompt the user for input until a specific condition is met. Here's an example:
password = ""
while password != "secret":
password = input("Enter the password: ")
print("Access granted!")
In this example, the while loop continues as long as the value of "password" is not equal to "secret". Inside the loop, the user is prompted to enter a password using the input() function. If the entered password is not "secret", the loop repeats the prompt. Once the user enters the correct password, the loop terminates, and "Access granted!" is printed.
Conclusion
The while loop is a powerful construct in Python that allows you to repeat a block of code until a certain condition is false. By understanding the syntax and usage of the while loop, you can create dynamic and interactive programs that perform repetitive tasks efficiently.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses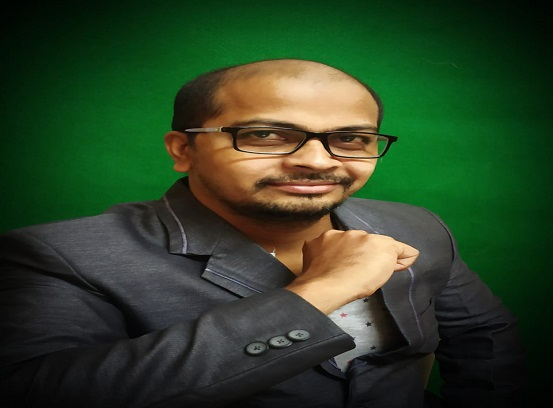
Most Popular Course topics
These are the most popular course topics among Software Courses for learners