Hi there, we’re Harisystems
"Unlock your potential and soar to new heights with our exclusive online courses! Ignite your passion, acquire valuable skills, and embrace limitless possibilities. Don't miss out on our limited-time sale - invest in yourself today and embark on a journey of personal and professional growth. Enroll now and shape your future with knowledge that lasts a lifetime!".
For corporate trainings, projects, and real world experience reach us. We believe that education should be accessible to all, regardless of geographical location or background.
1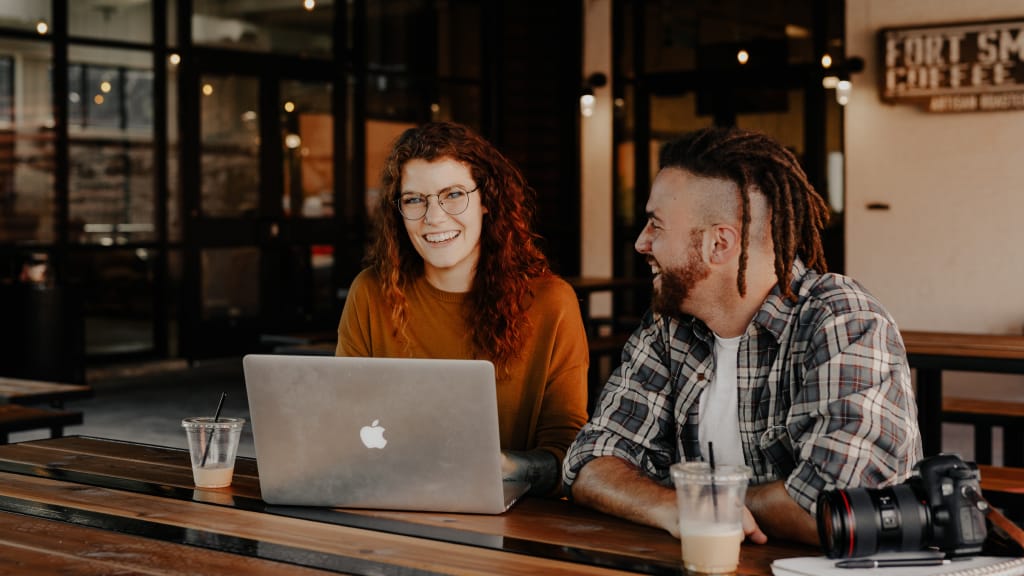
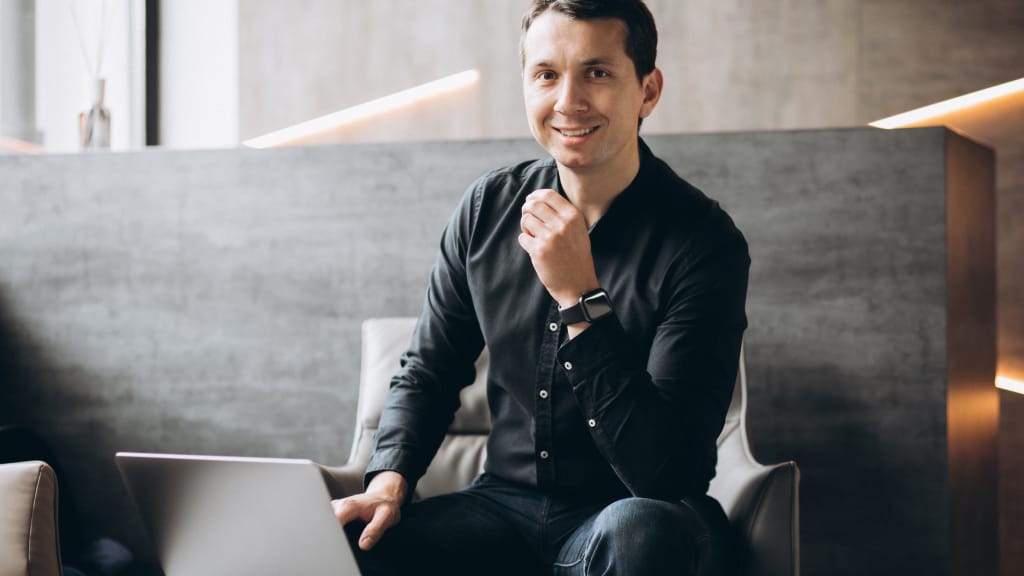
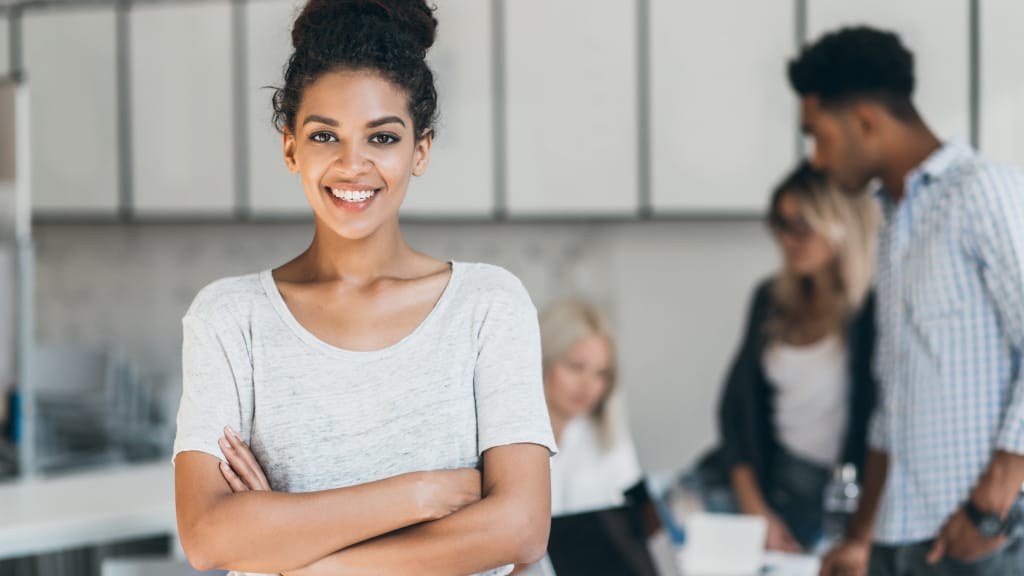
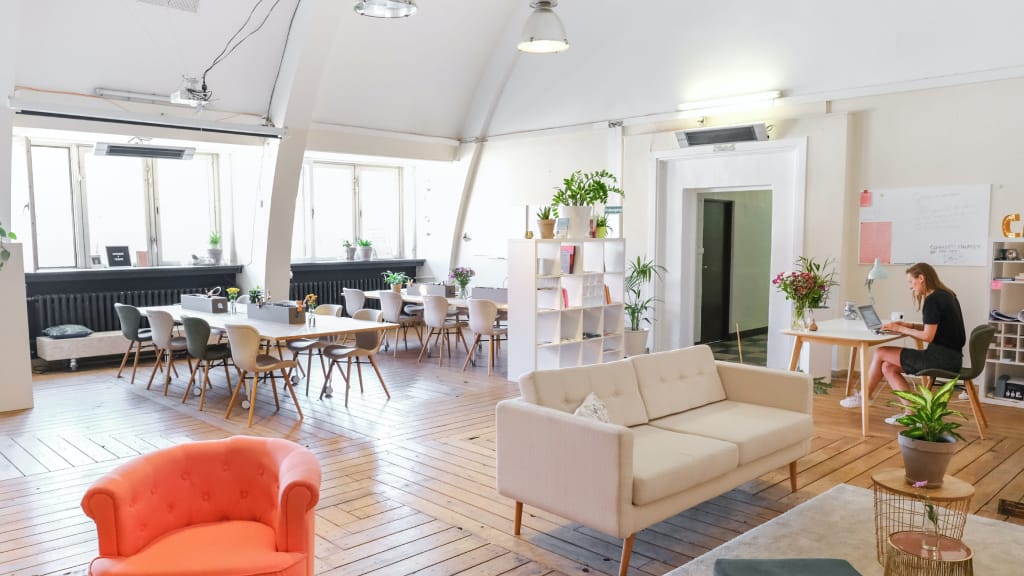
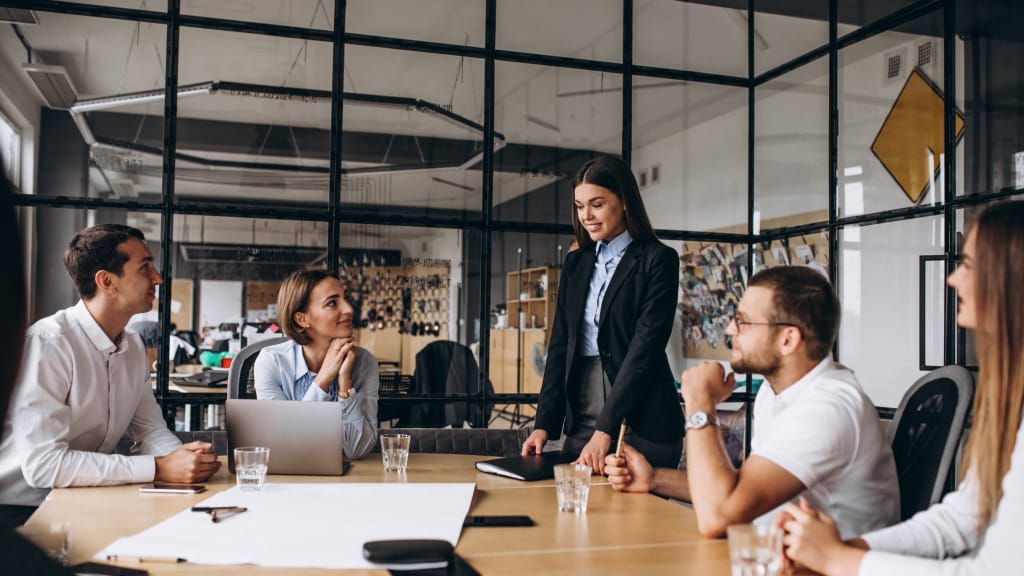
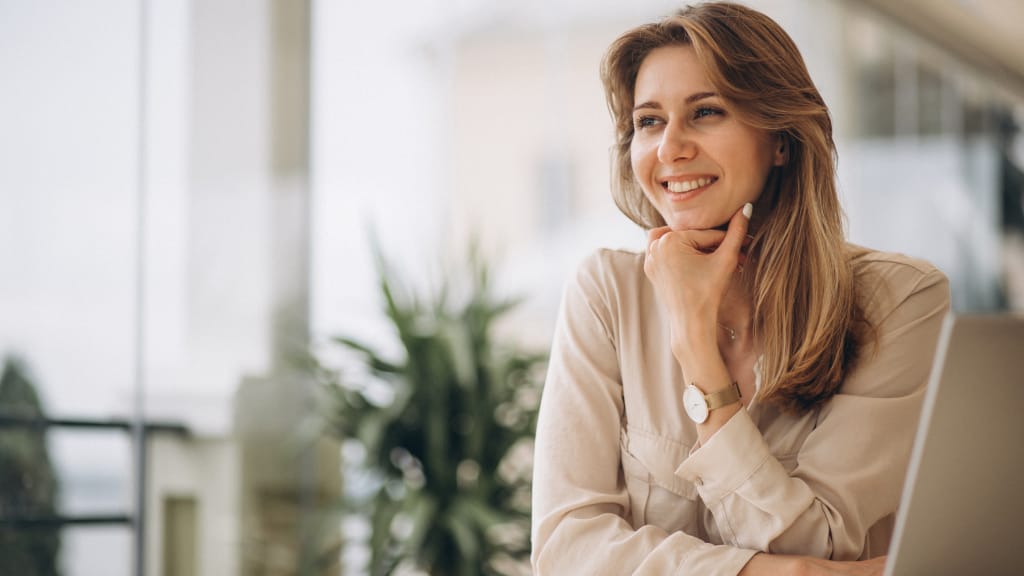
JavaScript Operators: Explained with Examples
Operators are essential components of JavaScript that allow you to perform various operations on data. They are used to manipulate values, compare values, perform mathematical calculations, and more. In this article, we will explore the different types of JavaScript operators and provide examples of their usage.
Arithmetic Operators
Arithmetic operators are used to perform mathematical calculations. Here are some common arithmetic operators in JavaScript:
+
(Addition): Adds two values together.-
(Subtraction): Subtracts one value from another.*
(Multiplication): Multiplies two values./
(Division): Divides one value by another.%
(Modulo): Returns the remainder of a division.++
(Increment): Increases the value by 1.--
(Decrement): Decreases the value by 1.
Here's an example that demonstrates the usage of arithmetic operators:
let x = 5;
let y = 2;
let addition = x + y; // 7
let subtraction = x - y; // 3
let multiplication = x * y; // 10
let division = x / y; // 2.5
let modulo = x % y; // 1
x++; // 6
y--; // 1
Comparison Operators
Comparison operators are used to compare values and determine the relationship between them. They return a boolean value (true or false) based on the comparison result. Here are some common comparison operators in JavaScript:
==
(Equality): Checks if two values are equal.!=
(Inequality): Checks if two values are not equal.===
(Strict Equality): Checks if two values are equal and of the same type.!==
(Strict Inequality): Checks if two values are not equal or not of the same type.>
(Greater Than): Checks if the left value is greater than the right value.<
(Less Than): Checks if the left value is less than the right value.>=
(Greater Than or Equal To): Checks if the left value is greater than or equal to the right value.<=
(Less Than or Equal To): Checks if the left value is less than or equal to the right value.
Here's an example that demonstrates the usage of comparison operators:
let x = 5;
let y = 2;
console.log(x == y); // false
console.log(x != y);
// true
console.log(x === '5'); // false
console.log(x !== '5'); // true
console.log(x > y); // true
console.log(x < y); // false
console.log(x >= 5); // true
console.log(y <= 1); // false
Logical Operators
Logical operators are used to combine or manipulate boolean values. They allow you to perform logical operations and make decisions based on multiple conditions. Here are the three common logical operators in JavaScript:
&&
(Logical AND): Returns true if both operands are true.||
(Logical OR): Returns true if at least one operand is true.!
(Logical NOT): Inverts the boolean value of the operand.
Here's an example that demonstrates the usage of logical operators:
let x = 5;
let y = 2;
let z = 8;
console.log(x > y && y < z); // true
console.log(x > y || y > z); // true
console.log(!(x > y)); // false
Assignment Operators
Assignment operators are used to assign values to variables. They provide a shorthand way to perform operations and assign the result to a variable. Here are some common assignment operators in JavaScript:
=
(Assignment): Assigns a value to a variable.+=
(Add and Assign): Adds a value to a variable and assigns the result.-=
(Subtract and Assign): Subtracts a value from a variable and assigns the result.*=
(Multiply and Assign): Multiplies a variable by a value and assigns the result./=
(Divide and Assign): Divides a variable by a value and assigns the result.%=
(Modulo and Assign): Calculates the remainder and assigns the result.
Here's an example that demonstrates the usage of assignment operators:
let x = 5;
x += 2; // Equivalent to x = x + 2, x is now 7
x -= 3; // Equivalent to x = x - 3, x is now 4
x *= 2; // Equivalent to x = x * 2, x is now 8
x /= 4; // Equivalent to x = x / 4, x is now 2
x %= 3; // Equivalent to x = x % 3, x is now 2 (remainder of 2)
Conclusion
JavaScript operators are powerful tools that enable you to perform a wide range of operations on values and make logical decisions in your code. In this article, we explored arithmetic operators for mathematical calculations, comparison operators for value comparisons, logical operators for logical operations, and assignment operators for shorthand variable assignments. Understanding and utilizing these operators effectively will enhance your ability to write dynamic and functional JavaScript code.
4.5L
Learners
20+
Instructors
50+
Courses
6.0L
Course enrollments
Future Trending Courses
When selecting, a course, Here are a few areas that are expected to be in demand in the future:.
Future Learning for all
If you’re passionate and ready to dive in, we’d love to join 1:1 classes for you. We’re committed to support our learners and professionals their development and well-being.
View Courses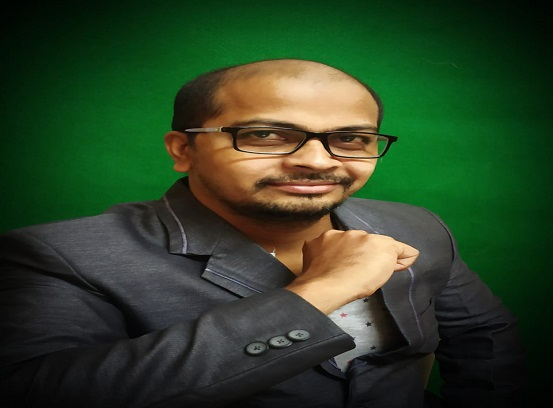
Most Popular Course topics
These are the most popular course topics among Software Courses for learners